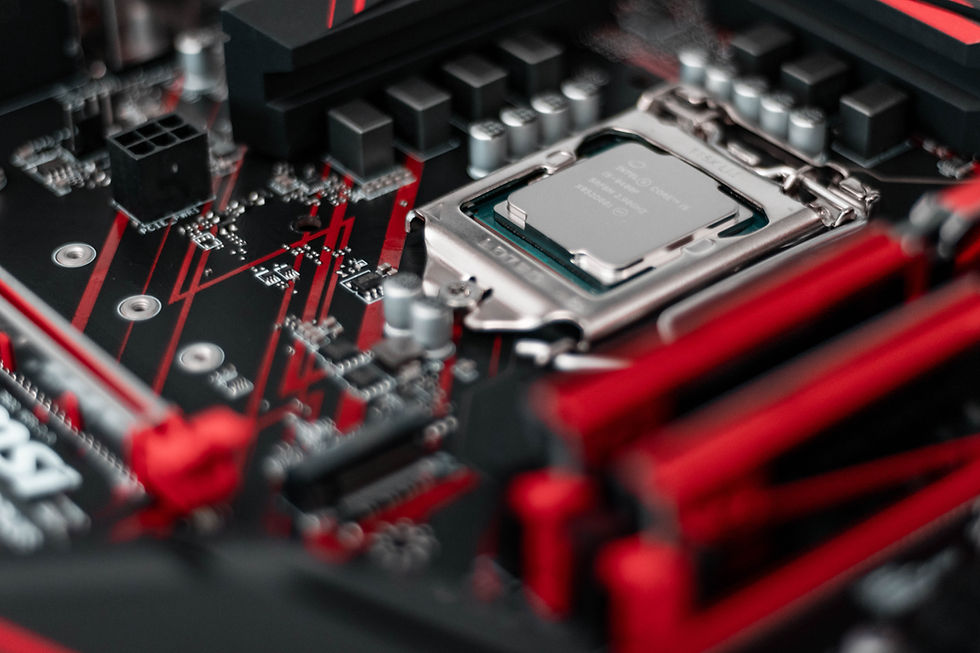
A REST API (Representational State Transfer API) is a way to allow communication between client and server applications using standard HTTP methods like GET, POST, PUT, DELETE, etc. It is stateless and relies on well-defined principles to organize and retrieve resources. REST defines how resources on the web are structured and accessed through HTTP requests.
Core Concepts of REST API
Resources:
Resources represent entities or objects (e.g., a user, product, or order).
Each resource is identified by a unique URL (Uniform Resource Locator).
Example:
/users - Represents all users.
/users/1 - Represents a specific user with ID 1.
HTTP Methods: REST APIs use standard HTTP methods to perform CRUD (Create, Read, Update, Delete) operations:
GET: Retrieve data from the server.
POST: Create new resources on the server.
PUT: Update an existing resource.
PATCH: Partially update an existing resource.
DELETE: Remove a resource.
Statelessness:
Each request from the client to the server must contain all the information necessary to process the request.
The server does not store the state of the client session between requests.
Representation:
Resources are typically represented in formats like JSON or XML.
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
}
Client-Server Separation:
The client (frontend) and the server (backend) are independent.
The server provides data through APIs, and the client is responsible for presenting it.
Uniform Interface: REST APIs follow a consistent structure for interacting with resources. This makes it easier for developers to understand and use the API.
Layered System:
REST APIs allow the use of additional layers (e.g., authentication, caching, load balancers) without affecting the client-server interaction.
Real-World Example: Online Food Delivery App
Resources:
Users: Represents customers and admins.
Restaurants: Represents restaurants providing food.
Orders: Represents customer orders.
Menu Items: Represents food items offered by restaurants.
HTTP Endpoints:
Resource | HTTP Method | Endpoint | Description |
Users | GET | /api/users | Fetch all users |
User (specific) | GET | /api/users/:id | Fetch details of a user |
Users | POST | /api/users | Create a new user |
Users | PUT | /api/users/:id | Update user details |
Users | DELETE | /api/users/:id | Delete a user |
Menu Items | GET | /api/menu | Fetch all menu items |
Menu Item (specific) | GET | /api/menu/:id | Fetch details of a menu item |
Orders | POST | /api/orders | Place a new order |
Orders (specific) | GET | /api/orders/:id | Fetch details of a specific order |
REST API Principles
Use Nouns, Not Verbs:
Use nouns to represent resources in the API endpoint.
Good: /api/users
Bad: /api/getUsers
HTTP Status Codes:
Use appropriate status codes for responses:
200 OK: Successful request.
201 Created: Resource successfully created.
400 Bad Request: Invalid request data.
404 Not Found: Resource not found.
500 Internal Server Error: Server error.
Versioning:
Use versioning to avoid breaking existing clients when the API changes.
Example: /api/v1/users
Filter, Sort, and Paginate:
Allow clients to filter, sort, and paginate results.
Example:
/api/menu?category=drinks
/api/menu?sort=price&order=asc
/api/menu?page=2&limit=10
Authentication and Authorization:
Protect sensitive resources using mechanisms like JWT (JSON Web Token) or OAuth.
Example:
Secure /api/orders so only authenticated users can access their orders.
Benefits of REST API
Scalability: Easy to scale because client and server are decoupled.
Flexibility: Can be used with any programming language or platform.
Simplicity: Follows a straightforward URL structure and HTTP methods.
Wide Adoption: Widely supported by modern frameworks and tools.
Tools for Building and Testing REST APIs
Node.js and Express.js: Build REST APIs efficiently.
Postman: Test API endpoints during development.
Swagger: Document APIs.
MongoDB: Store data for the API.
Comments