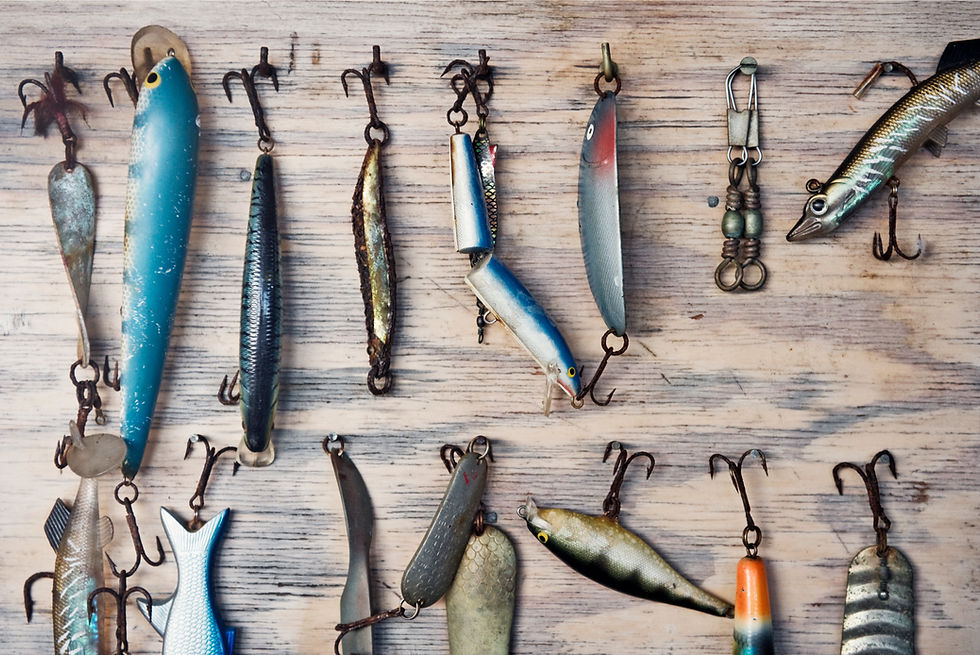
Hooks in React JS
Hooks in React JS are special functions that let you "hook into" React features like state and lifecycle methods in functional components. Introduced in React 16.8, hooks eliminate the need for class components, enabling developers to manage state, handle side effects, and build dynamic applications with functional components. Here are some of the most important React hooks:
Commonly Used Hooks
useState: Allows functional components to manage state.
useEffect: Handles side effects like API calls or DOM updates.
useContext: Provides access to React Context API values without prop drilling.
useRef: Accesses DOM elements or persistent variables without causing re-renders.
Understanding useState Hook in React with a Real-World Example
The useState hook is a React function that lets you add state to functional components. It allows you to track and update data that changes over time, such as user input, UI toggles, or counters. Syntax
const [state, setState] = useState(initialValue);
state: Holds the current state value.
setState: A function to update the state.
initialValue: The starting value of the state
Real-World Example: A Login Form
Imagine a login form where the user enters their email and password. The state tracks these inputs and displays a confirmation message when the user submits the form.
Code Example: Login Form Using useState:
import React, { useState } from 'react';
const LoginForm = () => {
const [email, setEmail] = useState(''); // State for email input
const [password, setPassword] = useState(''); // State for password input
const [message, setMessage] = useState(''); // State for confirmation message
const handleSubmit = (e) => {
e.preventDefault();
if (email && password) {
setMessage(`Welcome, ${email}!`);
} else {
setMessage('Please enter both email and password.');
}
};
return (
<div className="p-4 max-w-md mx-auto border rounded">
<h1 className="text-xl font-bold mb-4">Login</h1>
<form onSubmit={handleSubmit}>
<div className="mb-3">
<label className="block mb-1">Email:</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
className="border p-2 w-full"
placeholder="Enter your email"
/>
</div>
<div className="mb-3">
<label className="block mb-1">Password:</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
className="border p-2 w-full"
placeholder="Enter your password"
/>
</div>
<button
type="submit"
className="bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-600"
>
Login
</button>
</form>
{message && <p className="mt-4 text-green-500">{message}</p>}
</div>
);
};
export default LoginForm;
How It Works
Initialize State:
email and password states store the user’s inputs.
message state holds a confirmation message.
Update State:
The onChange handlers update the state as the user types.
React to State:
On form submission, the handleSubmit function checks the inputs and updates the message state.
Re-render UI:
When the state updates (e.g., message), React re-renders the component, displaying the appropriate feedback.
Benefits of useState
Simplifies state management in functional components.
Keeps components dynamic and interactive.
Triggers efficient re-renders when the state changes.
Comments