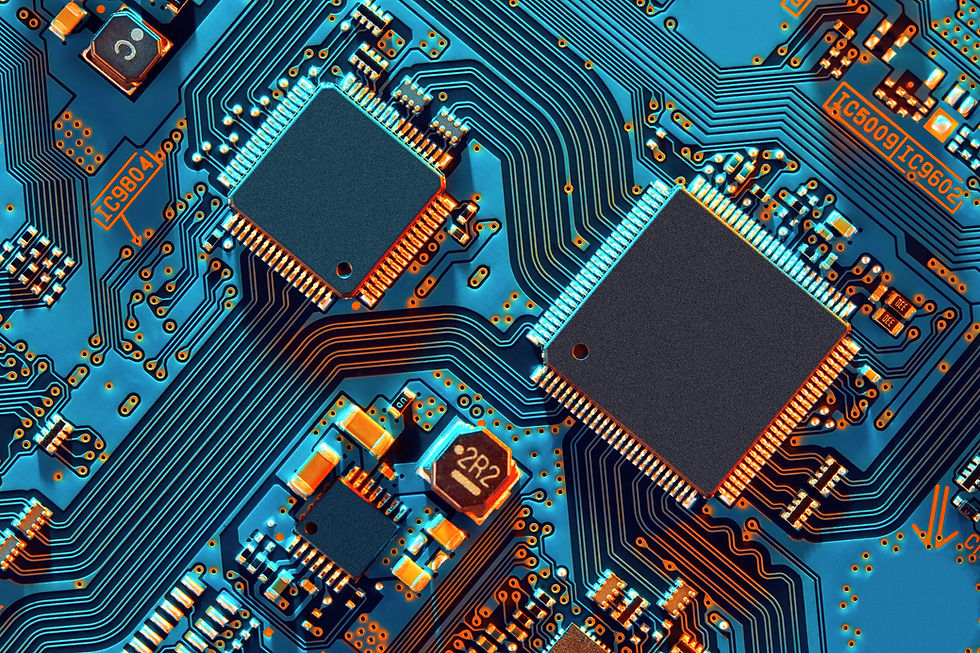
Using State in React
What is State in React?
In React, state refers to an object that holds dynamic data about a component. It determines how the component behaves and renders at a given time. Unlike props, which are immutable, state can change over time, allowing components to update dynamically without reloading the page.
State is managed within a component, and any change in state triggers a re-render, updating the UI to reflect the new data.
Examples for Using State in React: A To-Do List App
Imagine a to-do list application where users can add and remove tasks. The list of tasks is managed using React's state.
The state initially holds an empty array (no tasks).
When a user adds a new task, the state updates, and the UI re-renders to display the updated list.
Here’s how it works in code:
import React, { useState } from 'react';
const TodoApp = () => {
const [tasks, setTasks] = useState([]); // Initialize state with an empty array
const [input, setInput] = useState(''); // State for the input field
const addTask = () => {
if (input.trim()) {
setTasks([...tasks, input]); // Update the state with the new task
setInput(''); // Clear the input field
}
};
return (
<div className="p-4">
<h1 className="text-xl font-bold">To-Do List</h1>
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Enter a task"
className="border rounded p-2 w-full"
/>
<button
onClick={addTask}
className="bg-blue-500 text-white py-1 px-4 mt-2 rounded hover:bg-blue-600"
>
Add Task
</button>
<ul className="mt-4">
{tasks.map((task, index) => (
<li key={index} className="border-b py-1">{task}</li>
))}
</ul>
</div>
);
};
export default TodoApp;
How It Works:
State Initialization: The tasks state is initialized as an empty array.
Updating State: The addTask function adds the new task to the array by creating a new array with the existing tasks and the new one.
Re-rendering: When the state changes, React re-renders the Ul element to display the updated list of tasks.
Conclusion
Комментарии