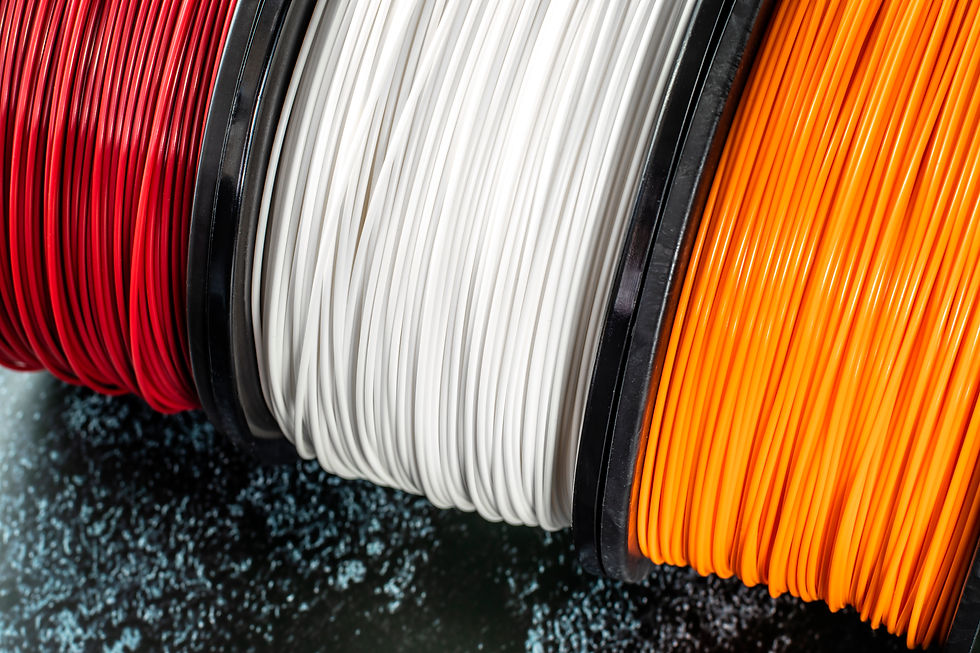
Unveiling the Magic of the JavaScript Spread Operator: Real World Examples and Use Cases
The JavaScript Spread Operator (`...`) is a feature introduced in ECMAScript 2015 (ES6) that gives developers a simple way to expand elements from an iterable—like arrays or strings—into individual elements. This feature may seem straightforward, but it brings about significant improvements in code simplicity and readability. In this post, we will explore the spread operator, its various applications, and provide real-world examples to illustrate its usefulness.
What is the Spread Operator in React?
The Spread Operator in React allows you to expand elements of an iterable into another iterable. For arrays, it enables easy concatenation without modifying the original arrays. It's also helpful for cloning objects and arrays, particularly important for preserving immutability in applications.
For instance, to concatenate two arrays, one might typically use:
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
let combined = array1.concat(array2);
With the spread operator, the same action becomes:
let combined = [...array1, ...array2];
The spread operator (...) creates a new array (combined) and spreads (...) / copies all elements from the existing arrays (array1 and array2) into this new array, effectively merging them. This approach not only makes the code cleaner but also makes it clear that you are merging the arrays.
Example: Adding Elements to an Array
The spread operator (...) creates a new array and spreads (...) / copies all existing primitive values into the newly created array.
const fruits = ["apple", "banana"];
const newFruits = [...fruits, "orange"];
console.log(newFruits); // ["apple", "banana", "orange"]
Keeps the original array intact while adding new elements!
How It Works?
Creates a new array (newFruits).
Spreads (...fruits) → Copies all elements ("apple", "banana") into the new array.
Adds "orange" at the end.
Since strings ("apple", "banana", "orange") are primitive values, they are copied by value (not reference).
Example: Copying & Merging Objects
The spread operator (...) creates a new object and spreads (...) / copies all existing object properties into the newly created object, while updating only the age property.
const user = { name: "Alice", age: 25 };
const updatedUser = { ...user, age: 26 };
console.log(updatedUser); // { name: "Alice", age: 26 }
Creates a new object (updatedUser).
Spreads (...user) → Copies all properties (name: "Alice", age: 25) into the new object.
Updates age: 26, overriding the original age: 25.
The original user object remains unchanged (immutability preserved).
Example: Function Arguments
The spread operator can also expand an array into separate arguments for function calls, allowing for flexible function definitions.
const numbers = [1, 2, 3, 4];
function add(a, b, c, d) {
return a + b + c + d;
}
console.log(add(...numbers)); // Output: 10
Here, the `numbers` array is conveniently passed as individual arguments to the `add` function.
Example: Removing Duplicates
Removing Duplicates with Set + Spread
const nums = [1, 2, 2, 3, 4, 4];
const uniqueNums = [...new Set(nums)];
console.log(uniqueNums); // [1, 2, 3, 4]
Uses Set to remove duplicates.
Uses ... to convert back to an array
Use Cases in Modern Frameworks
Many popular frameworks and libraries utilize the spread operator to streamline code and enhance efficiency. Here are key examples across frameworks:
React: The spread operator simplifies state and props management, especially with complex data structures.
Vue: Vue also employs the spread operator for efficient property merging during state updates.
Angular: In Angular, it is frequently used to combine multiple source objects, improving immutability management.
Final Thoughts
The JavaScript Spread Operator is a valuable tool that improves code clarity, efficiency, and maintainability. It enables developers to merge arrays, clone data, expand function arguments, and manage immutable state effectively.
Utilizing the spread operator can lead to cleaner, more reliable code that adheres to modern JavaScript practices. Whether you are an experienced developer or just starting out, incorporating the spread operator into your toolkit will significantly enhance your coding experience.
The next time you modify arrays or objects, remember the practicality and elegance of the JavaScript Spread Operator!
Comentários