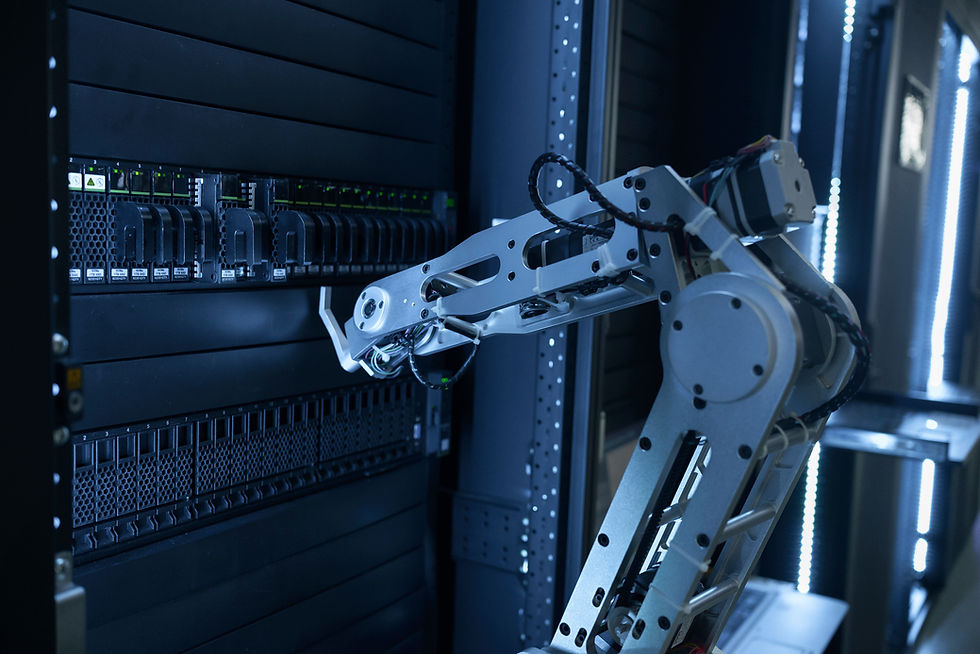
Basic Express Server
Express is a popular web framework for Node.js that simplifies the process of building web applications and APIs. Express provides a simple and consistent API for handling HTTP requests and responses, and includes a number of features and middleware that make it easy to handle common web development tasks, such as routing, authentication, and error handling.
Setting up a Project with Express
To use basic Express server in a Node.js project, you first need to install it using npm (Node Package Manager). You can do this by running the following command in your project directory:
> npm install express
This will install the latest version of Express in your project's node_modules directory.
Once you've installed Express, you can create a new Express application by requiring the express module and creating a new instance of the express class, like so:
const express = require('express');
const app = express();
To create routes in your Express application, you need to use the express.Router object. Here's an example of how to create a simple route that responds with a message:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, world!');
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In this example, we're creating a new Express application by calling the express() function. We're then creating a new route using the app.get() method, which specifies that this route should respond to HTTP GET requests. The first argument to app.get() is the URL path for the route, which in this case is '/' (i.e., the root URL). The second argument is a callback function that gets executed when a client requests this route. In this case, we're simply sending a plain text message back to the client using the res.send() method.
Finally, we're starting the Express server by calling the app.listen() method, which listens for incoming HTTP requests on port 3000.
Using Middleware
Middleware functions are functions that can be executed before or after the request/response process. They can be used to modify the request or response objects, or to execute additional code before or after the request is processed. Middleware functions can be registered using the app.use() method.
For example, the body-parser middleware can be used to parse incoming request bodies, such as JSON, URL-encoded, or multipart form data. Here's an example of how to use body-parser:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/users', (req, res) => {
const user = req.body;
// do something with the user object
res.send('User created');
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In this example, we're using the body-parser middleware to parse incoming JSON request bodies. We're then creating a new route that responds to HTTP POST requests on the /users URL path. The req.body object contains the parsed JSON object from the request body.
Using Routing
Express provides a flexible routing system that allows you to define URL paths and HTTP methods for your routes. You can use variables in the URL paths, and define multiple middleware functions for each route.
For example, here's how to define a route that responds to a dynamic URL path:
const express = require('express');
const app = express();
app.get('/users/:id', (req, res) => {
const id = req.params.id;
// do something with the id parameter
res.send(`User ${id}`);
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In this example, we're defining a new route that responds to HTTP GET requests on a URL path that includes a dynamic id parameter. The req.params object contains the parameters from the URL path.
You can define as many routes and middleware functions as you need in your Express application, and you can chain them together using the app.use() method. For example, you might use middleware functions to handle authentication, logging, or error handling, like so:
app.use(express.json()); // parse JSON request bodies
app.use(express.urlencoded({ extended: true })); // parse URL-encoded request bodies
app.use(logger('dev')); // log requests to the console
app.use('/api', apiRouter); // mount an API router at the /api URL
app.use(errorHandler); // handle errors in the application
In this example, we're using some built-in middleware functions provided by Express (express.json() and express.urlencoded()) to parse JSON and URL-encoded request bodies. We're also using the logger middleware to log requests to the console, and we're mounting an API router at the /api URL using the app.use() method. Finally, we're using a custom error-handling middleware function (errorHandler) to handle errors in the application.
Error handling
Express provides several ways to handle errors in your application, including built-in error handlers, custom error handlers, and middleware functions.
For example, you can define a custom error handler function that handles errors that occur during request processing:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
throw new Error('Something went wrong');
});
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong');
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In this example, we're defining a new route that throws an error when it's accessed. We're then defining a custom error handler middleware function using the app.use() method. This function logs the error to the console and sends a 500 status code and error message to the client.
Comments