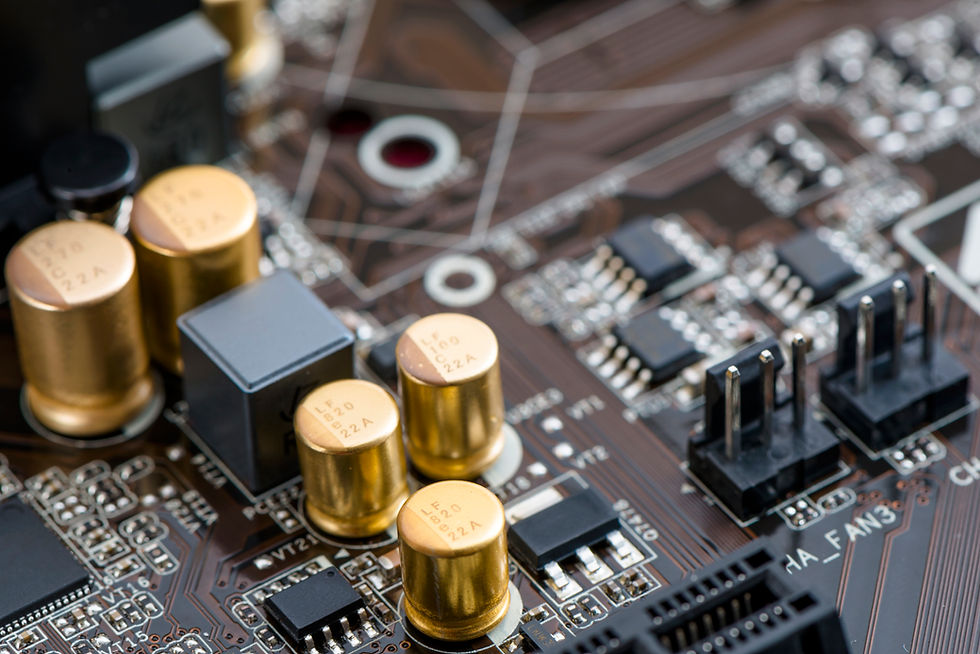
Definition
REST API: A REST API (Representational State Transfer API) is a standardized architecture and protocol for building web services that allow clients to interact with server-side resources (like data or functionalities) using HTTP methods (GET, POST, PUT, DELETE). It defines a set of rules for how the client-server interaction should occur.
Routes: In the context of web applications (e.g., Express.js), routes refer to the endpoints or paths defined within an application that determine how specific HTTP requests (like GET, POST) are handled. Routes are the building blocks of a REST API.
Scope
REST API: A REST API is a complete interface for accessing and manipulating resources. It encompasses routes, middleware, responses, and any additional rules or constraints required to adhere to RESTful principles.
Routes: Routes are a subset of the API implementation. They focus on mapping incoming HTTP requests to specific functions or logic in the server application.
Purpose
REST API:
Facilitates interaction between clients (like web browsers or mobile apps) and the server.
Provides a structured way to perform CRUD operations on resources.
Ensures standardization for scalability and interoperability.
Routes:
Handle specific requests and responses within the application.
Serve as the connection points between HTTP methods (like GET, POST) and application logic.
Example: REST API
Consider a REST API for managing a user resource.
Base URL: http://example.com/api/users
API Endpoints:
GET /api/users → Fetch all users.
POST /api/users → Create a new user.
PUT /api/users/:id → Update a user by ID.
DELETE /api/users/:id → Delete a user by ID.
Routes: The routes in the Express.js application that implement the REST API above could look like this:
const express = require('express');
const app = express();
app.get('/api/users', (req, res) => {
// Logic to fetch all users
res.send('Fetch all users');
});
app.post('/api/users', (req, res) => {
// Logic to create a new user
res.send('Create a new user');
});
app.put('/api/users/:id', (req, res) => {
// Logic to update a user by ID
res.send(`Update user with ID ${req.params.id}`);
});
app.delete('/api/users/:id', (req, res) => {
// Logic to delete a user by ID
res.send(`Delete user with ID ${req.params.id}`);
});
In this example:
The REST API provides the entire interface to manage "users."
The routes are individual handlers for specific HTTP methods and endpoints.
RESTful Constraints
REST API:
Follows RESTful principles like statelessness, resource-based URIs, and standardized HTTP methods.
Includes response standards such as returning HTTP status codes (200, 404, 500) and data in JSON/XML format.
Routes:
Do not inherently follow RESTful principles. They can exist in any form within a web application (e.g., /home, /dashboard).
Relationship
A REST API is built using routes as its core component.
Routes define the endpoints, and the REST API provides the overall design, architecture, and logic for using those routes in a standardized manner.
Key Differences at a Glance
Feature | REST API | Routes |
Definition | Interface for interacting with resources | Specific endpoints in the application |
Scope | Encompasses routes and additional rules | Focused on handling requests and responses |
Purpose | Enable client-server communication | Map HTTP requests to server logic |
Standardization | Follows REST principles | No inherent standardization |
コメント