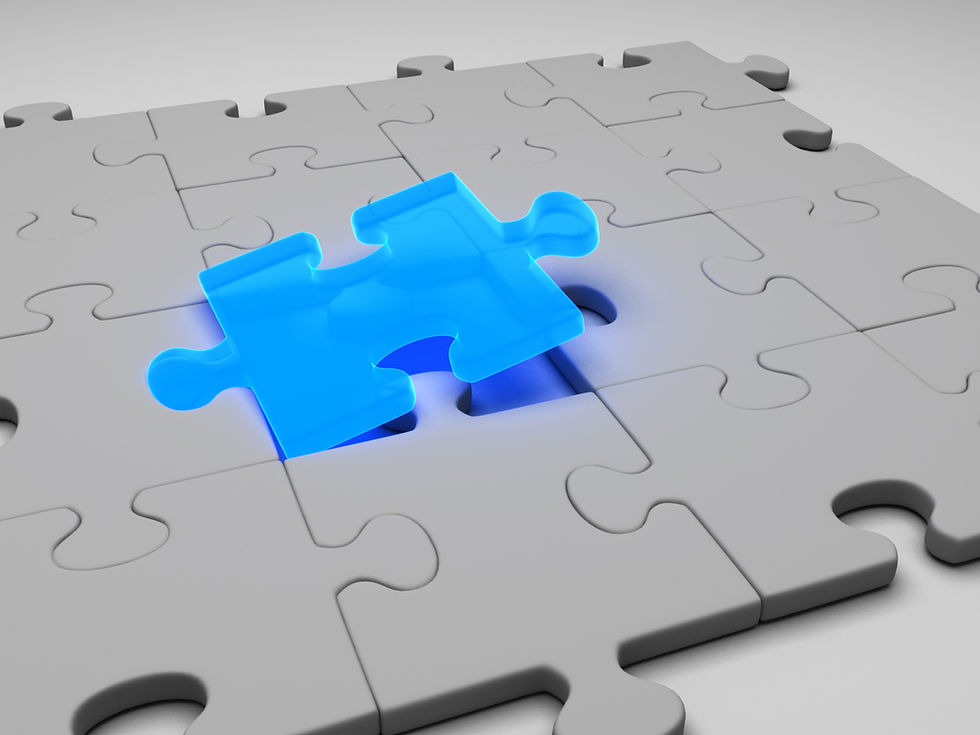
ReactJS JavaScript: The Puzzle of Modularity-Unraveling React's Component-Based Architecture
Building complex user interfaces (UIs) in web development can often feel overwhelming, much like assembling a challenging puzzle. Each piece needs to fit seamlessly with the others to create a cohesive design, yet must also function independently when necessary. Luckily, React’s modularity offers a powerful solution through its component-based architecture. This design approach allows developers to break down intricate applications into smaller, reusable components, leading to better organization, easier maintenance, and improved teamwork.
By applying the principles of component composition, developers can create self-contained components that function in various contexts. In this post, we will explore how React’s modularity simplifies UI development using a tangible example: a recipe application.
Understanding React’s Component-Based Architecture
ReactJS JavaScript: At its core, React is built around components, which are small, reusable pieces of code that represent part of the user interface. Each component can manage its own state and logic, making it a standalone unit. This modular design offers several benefits:
Reusability: Developers can use components across different parts of an application, saving time and minimizing code duplication. For example, a button component designed for a recipe app can also be used in a shopping cart app.
Maintainability: By dividing the application into smaller components, developers can address specific issues without affecting the entire codebase. In fact, studies show that modular code is 30% easier to maintain.
Collaboration: Teams can work on separate components simultaneously, which expedites the development process. According to a survey, 70% of developers believe that component-based architecture improves team collaboration.
Testing: Smaller units make it easier to conduct targeted testing, ensuring that each component performs as expected in isolation.
Understanding these principles sets the stage for exploring a real-world example that demonstrates how to effectively use React’s capabilities.
Real-World Example: A Recipe App
Let’s consider a recipe application where users can search, view, and save their favorite recipes. This type of app features complex UI elements, such as search interfaces, recipe cards, and favorites lists. Here’s how we can design this app using React’s component-based architecture.
Main Components
Search Bar: Positioned at the top of the app, this component allows users to input search queries by ingredients or recipe names.
Recipe List: This component fetches and displays recipes based on user input, showcasing the versatility of data handling in React.
Recipe Card: Each recipe is represented through a Recipe Card component, detailing essential information about the dish.
Favorites: Users can mark recipes as favorites, which are displayed in a dedicated Favorites component.
Recipe Detail: Clicking on a Recipe Card directs the user to a Recipe Detail component with cooking instructions and preparation time.
The Search Bar Component
The Search Bar component manages user input and triggers the recipe search. Here's a simplified version in React:
import React, { useState } from 'react';
const SearchBar = ({ onSearch }) => {
const [query, setQuery] = useState('');
const handleSearch = (event) => {
event.preventDefault();
onSearch(query);
};
return (
<form onSubmit={handleSearch}>
<input
type="text"
value={query}
onChange={(e) => setQuery(e.target.value)}
placeholder="Search recipes..."
/>
<button type="submit">Search</button>
</form>
);
};
export default SearchBar;
In this component, we maintain the internal state for user input and call the `onSearch` function supplied by the parent component.
Connecting Components
Next, we connect the Search Bar to the Recipe List in the main App component:
import React, { useState } from 'react';
import SearchBar from './SearchBar';
import RecipeList from './RecipeList';
const App = () => {
const [recipes, setRecipes] = useState([]);
const fetchRecipes = async (query) => {
const response = await fetch(`https://api.example.com/recipes?search=${query}`);
const data = await response.json();
setRecipes(data.recipes);
};
return (
<div>
<h1>Recipe Finder</h1>
<SearchBar onSearch={fetchRecipes} />
<RecipeList recipes={recipes} />
</div>
);
};
export default App;
In this code, the App component manages the overall application state while passing necessary data and functions to child components. This demonstrates how component composition works, allowing higher-order components to manage their children effectively.
The Recipe List Component
The Recipe List component displays a list of Recipe Cards based on the data it receives. Each recipe can now be represented with an individual Recipe Card component:
import React from 'react';
import RecipeCard from './RecipeCard';
const RecipeList = ({ recipes }) => {
return (
<div>
{recipes.map(recipe => (
<RecipeCard key={recipe.id} recipe={recipe} />
))}
</div>
);
};
export default RecipeList;
Recipe Card Component
The Recipe Card showcases recipe details and allows users to save favorites. Here’s the setup:
import React from 'react';
const RecipeCard = ({ recipe }) => {
return (
<div className="recipe-card">
<h3>{recipe.title}</h3>
<p>{recipe.description}</p>
<button>Add to Favorites</button>
</div>
);
};
export default RecipeCard;
With each Recipe Card, users can view details and save their selections, simplifying interactions.
Advantages of Component-Based Architecture
Separation of Concerns: Each component takes care of a specific functionality like searching or displaying recipes, creating clarity and simplicity.
Ease of Updates: If changes are needed in the search functionality or design of Recipe Cards, developers can adjust those particular components without affecting other parts.
Enhanced Collaboration: With well-defined components, team members can develop the Recipe List and Recipe Card in tandem, improving overall efficiency.
Scalability: Adding features like user login or filtering options can be done easily with new, separate components, allowing the app to grow organically.
Wrapping it Up
React’s modularity really stands out through its component-based architecture, empowering developers to break down complex interfaces into reusable and independent components. Using our recipe app example, the process of managing components is clearly illustrated.
This approach simplifies development, improves maintainability, and enhances team collaboration. As web application demands increase, mastering React’s component-based architecture remains an essential skill for developers. By piecing together each component, developers can create intricate UIs that fit perfectly together to form a seamless user experience.
Comments