ReactJS Functional Component Example
- coding z2m
- Dec 9, 2024
- 4 min read
Updated: Feb 6
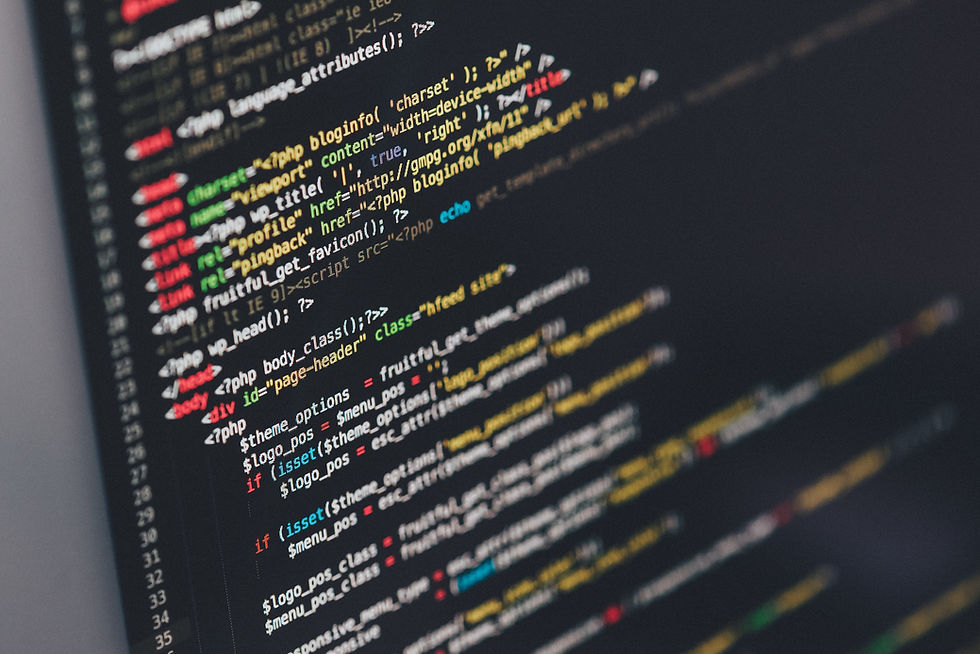
Unleashing the Power of ReactJS Functional Component Example: Building Dynamic UIs in React Like a Pro
In the fast-paced world of web development, React shines as a premier library for creating interactive user interfaces. A cornerstone of this flexibility is the use of components. Functional components, in particular, have become a favorite among developers due to their simplicity and effectiveness in crafting engaging user experiences. This article will examine how to harness functional components to build dynamic and interactive user interfaces within React applications.
Component Reusability
ReactJS Functional Component Example: One of the standout features of functional components is their reusability. By breaking down a user interface into smaller pieces, developers can easily apply these components throughout their applications. This approach not only conserves time but also enhances maintainability.
For example, think of a button component used in multiple parts of an application. Instead of rewriting identical code for the button in various files, you can create a single `Button` functional component and import it wherever needed.
const Button = ({ label, onClick }) => {
return <button onClick={onClick}>{label}</button>;
};
This `Button` component can now be reused across the application by simply passing different `label` and `onClick` props as required.
To illustrate further, consider a scenario in a social media app where a `Like` button is used across several posts. Instead of implementing the button code in each post, you can just call the `Button` component, making updates simpler. When you want to change the styling or behavior of the button, you only need to do it in one place, ensuring consistency and reducing the chance of bugs.
ReactJS Functional components Example: In React are simple JavaScript functions that return JSX (JavaScript XML) to describe the UI. They are one of the two main types of components in React, the other being class components.
Key Features of Functional Components:
Simple and Readable: They are plain JavaScript functions, making them easier to read and understand compared to class components.
Stateless or Stateful: Initially, functional components were stateless, but with the introduction of React Hooks, they can now handle state and side effects.
Performance: Functional components are generally faster and consume fewer resources than class components, especially when React's optimization techniques (like memoization) are used.
Hooks Support: They leverage React Hooks (like useState, useEffect, etc.) to manage state, lifecycle, and other features.
Functional Components in React with Real-World Examples
Functional components in React are JavaScript functions that define how a user interface should appear. They are widely used due to their simplicity, performance, and modern features like React Hooks.
Let’s understand functional components better through real-world examples.
Example 1: Greeting Card Application
In a greeting card app, you might want to display a personalized message for users.
Component Code:
function GreetingCard({ recipientName, message }) {
return (
<div style={{ border: '1px solid #ccc', padding: '10px', margin: '10px' }}>
<h2>Dear {recipientName},</h2>
<p>{message}</p>
</div>
);
}
// Usage
<GreetingCard recipientName="John" message="Wishing you a wonderful day!" />
<GreetingCard recipientName="Emily" message="Happy Birthday! Enjoy your special day!" />
Real-World Use:
A greeting card website where users can customize messages for their friends or family.
Example 2: Product List in an E-commerce Store
An online store displays a list of products with details like name, price, and availability.
Component Code:
function ProductCard({ productName, price, isAvailable }) {
return (
<div style={{ border: '1px solid #ccc', padding: '15px', margin: '10px' }}>
<h3>{productName}</h3>
<p>Price: ${price.toFixed(2)}</p>
<p>{isAvailable ? "In Stock" : "Out of Stock"}</p>
</div>
);
}
// Usage
<ProductCard productName="Laptop" price={999.99} isAvailable={true} />
<ProductCard productName="Headphones" price={199.99} isAvailable={false} />
Real-World Use:
An e-commerce application displaying products with dynamic availability and pricing.
Example 3: Task Manager Application
A task manager app shows a list of tasks and allows users to mark tasks as completed.
Component Code:
import React, { useState } from 'react';
function TaskItem({ taskName }) {
const [isCompleted, setIsCompleted] = useState(false);
return (
<div
style={{
textDecoration: isCompleted ? 'line-through' : 'none',
margin: '5px 0',
}}
>
<input
type="checkbox"
checked={isCompleted}
onChange={() => setIsCompleted(!isCompleted)}
/>
{taskName}
</div>
);
}
// Usage
<TaskItem taskName="Buy groceries" />
<TaskItem taskName="Complete React project" />
Real-World Use:
A to-do list application allowing users to track and update their tasks.
Example 4: Dynamic Weather App
A weather app displays the current weather conditions for a given location.
Component Code:
function WeatherCard({ location, temperature, condition }) {
return (
<div style={{ padding: '10px', border: '1px solid #ccc', width: '200px' }}>
<h3>{location}</h3>
<p>Temperature: {temperature}°C</p>
<p>Condition: {condition}</p>
</div>
);
}
// Usage
<WeatherCard location="New York" temperature={25} condition="Sunny" />
<WeatherCard location="London" temperature={15} condition="Cloudy" />
Real-World Use:
A weather dashboard displaying live weather updates for multiple cities.
Example 5: Online Voting System
An online voting system displays options and tracks the number of votes each option receives.
Component Code:
import React, { useState } from 'react';
function VotingOption({ optionName }) {
const [votes, setVotes] = useState(0);
return (
<div style={{ margin: '10px 0' }}>
<p>{optionName}</p>
<button onClick={() => setVotes(votes + 1)}>Vote</button>
<p>Votes: {votes}</p>
</div>
);
}
// Usage
<VotingOption optionName="Option A" />
<VotingOption optionName="Option B" />
Real-World Use:
Polling platforms where users vote for their favorite options in real-time.
Why Functional Components Work Well in Real-World Apps
Reusability: These components can be reused with different props, reducing redundancy.
Hooks Support: Managing dynamic behaviors (like state and side effects) is simplified with React Hooks.
Scalability: Functional components are easier to maintain and scale as your application grows.
Performance: They are lightweight and efficient.
Conclusion
Functional components provide a modern, clean, and effective way to build UIs for various real-world scenarios, from e-commerce and task management to weather apps and voting systems. By leveraging React Hooks, they can handle stateful logic, making them as powerful as class components while being easier to write and maintain.
Comments