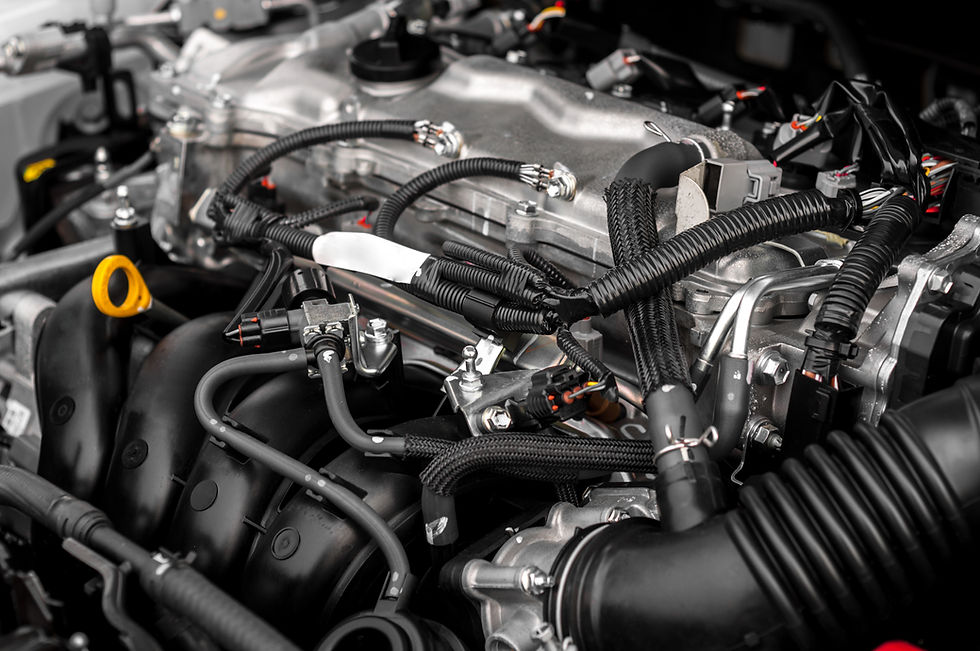
Props in React JS
What are Props in React.js?
Props in React JS (short for properties) are a way to pass data from a parent component to a child component in React. They allow components to be dynamic and reusable by providing inputs in the form of arguments. Props are immutable, meaning they cannot be changed by the child component that receives them.
Real-World Example: A Business Card Generator
Imagine you're creating a business card generator app. Each card should display unique details like the name, job title, and contact information. Instead of hardcoding this information, we use props to make the Business Card component reusable for multiple users.
Parent Component:
const App = () => {
return (
<div>
<BusinessCard
name="John Doe"
title="Software Engineer"
email="john.doe@example.com"
/>
<BusinessCard
name="Jane Smith"
title="Graphic Designer"
email="jane.smith@example.com"
/>
</div>
);
};
Child Component (Receiving Props):
const BusinessCard = ({ name, title, email }) => {
return (
<div className="business-card">
<h2>{name}</h2>
<p>{title}</p>
<a href={`mailto:${email}`}>{email}</a>
</div>
);
};
How Props Work in the Example:
Passing Data (Parent to Child):
The App component (parent) passes data like name, title, and email as props to the BusinessCard component.
This makes the BusinessCard reusable for different users.
Accessing Props:
The child component (BusinessCard) receives the data as an object and uses destructuring to access specific properties like name, title, and email.
These props are dynamically rendered within the JSX using curly braces {}.
Reusability:
By simply changing the values of props in the parent component, you can create multiple unique business cards without modifying the child component's logic.
Why Use Props?
Dynamic Rendering: Enables components to adapt based on the data they receive.
Reusability: Write a component once and use it with different inputs.
Separation of Concerns: Keeps components clean by separating data management (parent) from UI rendering (child).
Comentários