Online React JS Training
- coding z2m
- Jan 30
- 4 min read
Updated: Jan 31
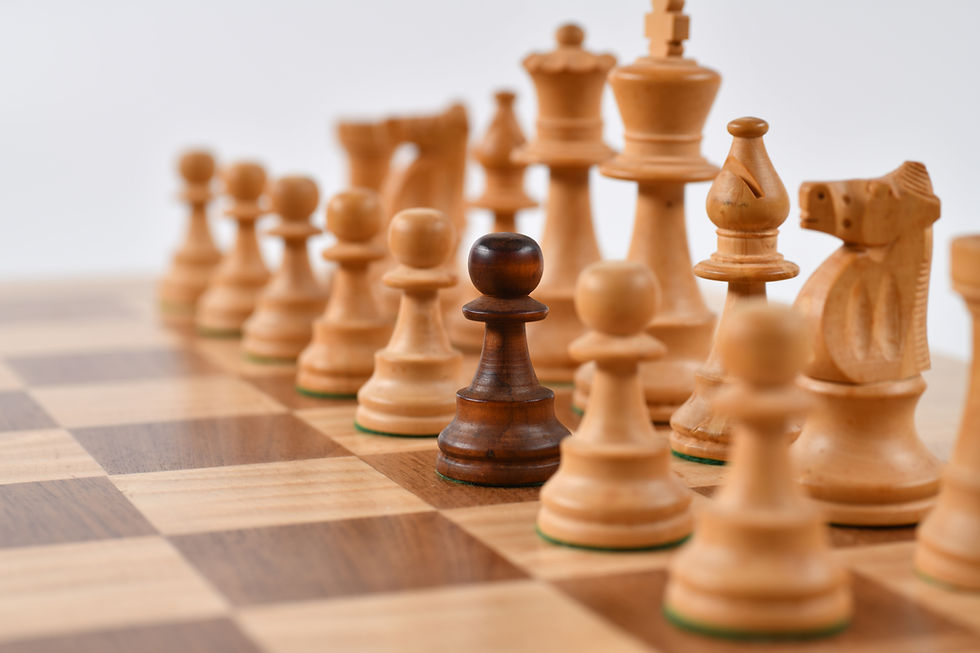
Online React JS Training: How React's Conditional Rendering, Hooks, Props & State Intertwine for Dynamic UIs
Online React JS Training: React JavaScript Framework has transformed how developers create user interfaces. By mastering its core features, you can design dynamic and responsive applications. Key components like conditional rendering, hooks, props, and state are vital for this process. In this post, we will examine how these features work together using a detailed example of an interactive recipe book.
Understanding the Basics
Before diving into the recipe book example, let’s quickly review the essential concepts we’ll explore.
Conditional Rendering
Conditional rendering enables components to behave differently based on specific conditions. This can be handy for toggling visibility or altering the styling of components in response to user actions. For instance, if a user checks off that they have tomatoes, the display might change to highlight recipes that use tomatoes.
Props
Props, short for properties, serve as a means for communication between components. They allow parent components to send data and behavior down to child components. For example, a `RecipeList` component might receive a list of ingredients as props to determine which recipes to display.
State
State is a built-in object in React used to manage component data that changes over time. When state updates, it triggers a re-rendering of the component, making it responsive to user actions. An example would be tracking how many ingredients are marked as available in our recipe book.
Hooks
React hooks are functions that allow developers to use state and lifecycle features in function components. Hooks like `useState` enable more flexible state management without the need for class components. For instance, `useState` can maintain the list of available ingredients in our application.
Real-World Example: Interactive Recipe Book
Let's create an interactive recipe book application. Users will be able to toggle ingredient availability and see relevant recipes based on what they have at home.
Setting Up Our Project
To start, create a new React application using Create React App:
npx create-react-app interactive-recipe-book
cd interactive-recipe-book
Next, we’ll construct our main components: `IngredientList` and `RecipeList`.
Creating the Ingredient List
Here, we will build an `IngredientList` component where users can indicate which ingredients they have:
import React, { useState } from 'react';
const IngredientList = ({ ingredients, toggleAvailability }) => {
return (
<ul>
{ingredients.map((ingredient) => (
<li key={ingredient.id}>
<input
type="checkbox"
checked={ingredient.available}
onChange={() => toggleAvailability(ingredient.id)}
/>
{ingredient.name}
</li>
))}
</ul>
);
};
In this code, users can mark ingredients as available. The `toggleAvailability` function updates the state based on user actions.
Managing State in the Parent Component
const App = () => {
const [ingredients, setIngredients] = useState([
{ id: 1, name: "Tomatoes", available: false },
{ id: 2, name: "Basil", available: false },
{ id: 3, name: "Olive Oil", available: false }
]);
const toggleAvailability = (id) => {
setIngredients(ingredients.map(ingredient =>
ingredient.id === id ? { ...ingredient, available: !ingredient.available } : ingredient
));
};
return (
<div>
<h1>Interactive Recipe Book</h1>
<IngredientList ingredients={ingredients} toggleAvailability={toggleAvailability} />
</div>
);
};
This code initializes the ingredient state and allows its availability status to be toggled.
Introducing Conditional Rendering with Recipe List
Now we will create the `RecipeList` component to display recipes based on the available ingredients:
const RecipeList = ({ ingredients }) => {
const recipes = [
{ id: 1, name: "Caprese Salad", requirements: [1, 2] },
{ id: 2, name: "Pasta with Olive Oil", requirements: [3] }
];
const availableRecipes = recipes.filter(recipe =>
recipe.requirements.every(req => ingredients.find(ing => ing.id === req && ing.available))
);
return (
<div>
<h2>Your Recipes</h2>
{availableRecipes.length > 0 ? (
<ul>
{availableRecipes.map(recipe => (
<li key={recipe.id}>{recipe.name}</li>
))}
</ul>
) : (
<p>No available recipes based on your ingredients.</p>
)}
</div>
);
};
The `RecipeList` component examines the available ingredients and displays corresponding recipes. If no recipes exist, it lets the user know.
Integrating Everything in the App Component
We will now merge the `IngredientList` and `RecipeList` components:
return (
<div>
<h1>Interactive Recipe Book</h1>
<IngredientList ingredients={ingredients} toggleAvailability={toggleAvailability} />
<RecipeList ingredients={ingredients} />
</div>
);
Now, our application allows users to check ingredient availability and receive recipe suggestions accordingly.
Benefits of This Approach
Separation of Concerns: By utilizing props to manage data and functionality, our code is cleaner and easier to maintain.
Responsive User Interface: State and conditional rendering create an interactive experience, instantly updating suggestions as ingredients change.
Reusable Components: Components can be tested and reused independently, supporting effective development practices.
Wrapping Up
In this post, we explored how React components like conditional rendering, hooks, props, and state work together. By building an interactive recipe book, we demonstrated the immediate feedback users receive based on their actions. This example illustrates how understanding these advanced techniques can empower developers to create engaging applications.
When constructing your next React project, remember the potential of combining these features. Each component you design can contribute to a responsive and user-friendly interface, making the development journey enjoyable and efficient.
Comments