JavaScript Event Loop
- coding z2m
- Nov 19, 2024
- 4 min read
Updated: Jan 27
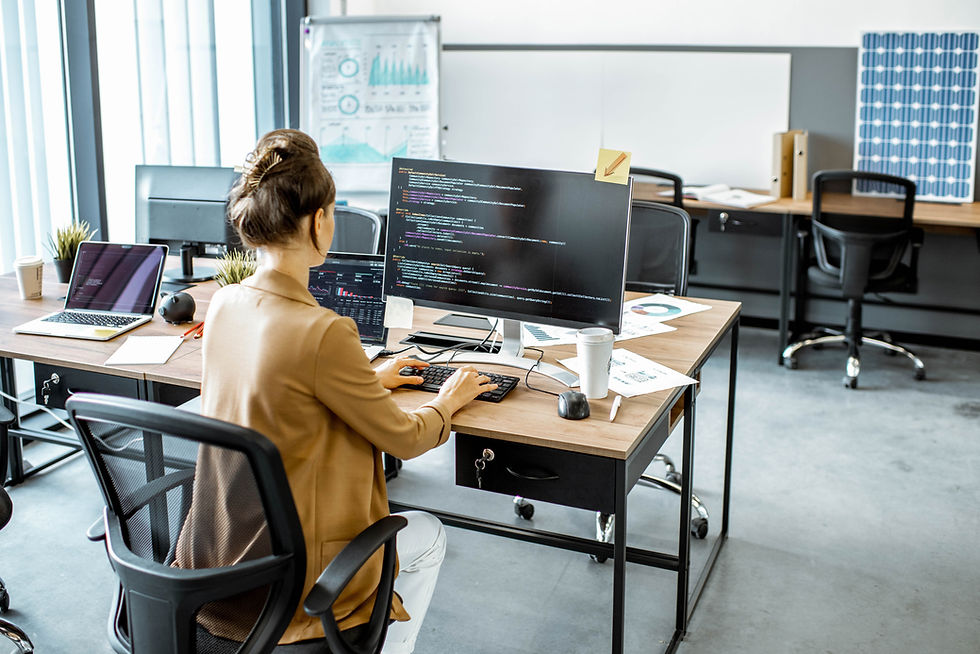
How Node.js's Event-Driven, Non-Blocking I/O Model Makes It Highly Efficient and Scalable: JavaScript Event Loop
Node.js is designed to handle a large number of concurrent requests efficiently, and its event-driven, non-blocking I/O model is at the heart of this capability. Here's an explanation of how these concepts contribute to Node.js's performance and scalability:
1. Event-Driven Architecture
In Node.js, the event-driven model means that operations such as reading files, making network requests, or querying databases don't block the execution of other code. Instead of waiting for these I/O operations to complete, Node.js uses an event loop to manage and handle multiple requests concurrently.
When an I/O operation (like reading a file or querying a database) is initiated, Node.js sends the operation off to the system's resources (like the OS or a database).
Instead of sitting idle and waiting for that operation to complete, Node.js continues to execute other tasks and responds when the I/O operation finishes, typically by emitting an event.
These events are handled by callback functions or Promises, which are invoked once the I/O task is complete, allowing the application to process the next operation.
This event-driven behavior allows Node.js to handle many requests at once without being blocked by slow I/O operations (such as file reads, network requests, or database queries).
2. Non-Blocking I/O
One of the key features of Node.js is that its I/O operations are non-blocking. This is the opposite of a traditional blocking I/O model, where a process would wait (or block) until a task, such as reading a file or waiting for a database query, is complete before moving on to the next operation.
In a blocking I/O model (like in many traditional server frameworks), if one request is waiting for data from a database or a file system operation, the entire thread or process is blocked. This means that no other tasks can be processed until that operation is complete, leading to inefficient use of system resources, especially under high traffic.
In contrast, Node.js doesn't block. When an I/O operation is called (like fs.readFile), Node.js sends it off to the system and continues executing other code. The main thread of Node.js, known as the event loop, remains free to handle other incoming requests, and once the I/O operation finishes, it triggers a callback to handle the result.
This non-blocking behavior ensures that Node.js can serve many requests concurrently without needing to spawn multiple threads or processes, making it more efficient than blocking, multi-threaded models for certain types of workloads.
3. The Event Loop
At the core of Node.js's event-driven(JavaScript Event Loop), non-blocking model is the event loop, which is responsible for handling asynchronous operations.
Here’s a simplified breakdown of how the event loop works:
Initial Execution: When a Node.js application starts, it executes the synchronous code first (such as setting up routes, initializing variables, etc.).
Asynchronous Tasks: Any asynchronous tasks (like network requests, file reads, or database queries) are sent off to the appropriate system resources, and callbacks or events are registered.
Event Loop: The event loop constantly checks whether there are any completed asynchronous tasks. If there are, it processes the corresponding callback functions and handles the results. If not, the event loop continues running, checking for new tasks.
The event loop operates in a single thread, which is why Node.js is often referred to as "single-threaded." However, it is capable of handling many concurrent requests because, instead of blocking while waiting for I/O operations, it uses the event loop to manage and process those requests asynchronously.
4. Scalability
The combination of event-driven and non-blocking architecture in Node.js makes it highly scalable:
Handling Concurrent Connections: Since Node.js doesn't wait for I/O operations to complete before moving on to other tasks, it can handle a massive number of concurrent connections with a single thread. This makes it ideal for I/O-heavy applications like real-time chat apps, APIs, and web servers.
Resource Efficiency: Unlike traditional multi-threaded servers that create new threads for each connection (which consumes significant system resources), Node.js can handle thousands of connections on a single thread. This reduces overhead and improves system resource utilization.
Load Balancing: In a production environment, Node.js can be paired with load balancing techniques (using tools like Nginx or PM2) to distribute the load across multiple CPU cores or servers, allowing even more scalability for high-traffic applications.
5. Example Use Case: Real-Time Applications
Consider a real-time application like a chat app. In a traditional server setup (using a multi-threaded server), each message might require a new thread to handle, leading to performance bottlenecks as the number of users grows.
However, with Node.js:
Each message can be handled by the event loop, allowing for millions of concurrent open connections (as in a real-time chat).
The event loop can continue running and processing other events (like sending messages or updating UIs) while waiting for other tasks (like network requests) to complete.
This results in much more efficient handling of concurrent users and messages without the need for excessive server resources.
6. Asynchronous APIs and Libraries
Node.js offers a wide range of asynchronous APIs and libraries built around its non-blocking model. Common I/O operations such as reading files (fs.readFile), querying a database, or making HTTP requests (http.get) are non-blocking by default. This makes it easier to build applications that can handle thousands (or even millions) of concurrent requests without excessive resource usage.
Summary:
Node.js's event-driven and non-blocking I/O model ensures that:
It can handle many concurrent connections and requests with a single thread.
It doesn’t block the execution while waiting for I/O operations to finish, making it highly efficient.
Its event loop enables the processing of multiple asynchronous tasks without needing multiple threads, thus optimizing performance and system resource usage.
These features make Node.js a perfect fit for I/O-heavy, real-time, and high-concurrency applications.
Comments