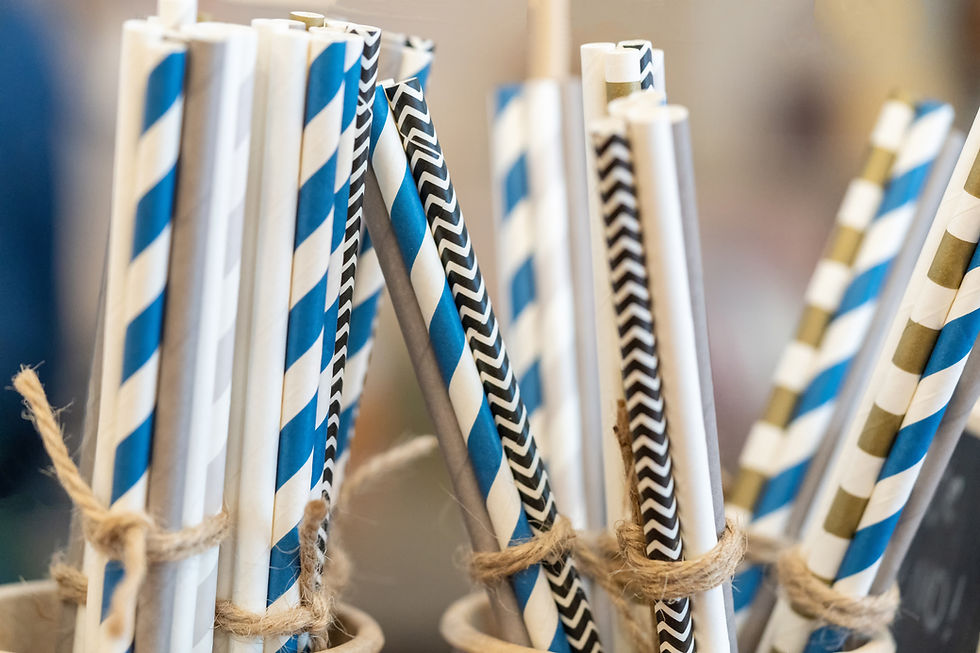
Understanding the Functional Update Pattern in React
In the fast-paced world of React development, effectively managing application state is essential. As developers aim to create fast and user-friendly interfaces, understanding state management patterns becomes vital. Among these patterns, the Functional Update Pattern emerges as a clear and effective method, especially when performing state updates based on previous values.
Let's take a closer look at the Functional Update Pattern, its significance, and how it can enhance the reliability of your components in React applications.
What is the Functional Update Pattern?
The Functional Update Pattern is a state management technique in React that allows developers to update state functionally. This method is particularly beneficial when new state values depend on previous ones. In React, state updates occur typically through the `useState` hook or the `setState` method in class components.
When implementing a functional update, you provide a function to your state updater. This function receives the previous state as an argument, which it then uses to return the new state. This approach guarantees that you're always working with the latest state, helping to avoid issues that arise from stale data.
Functional Updates React Example
Consider the following code snippet:
const [count, setCount] = useState(0);
const increment = () => {
setCount(prevCount => prevCount + 1);
};
In this Functional Updates React example, `setCount` is called with a function that accepts the previous count. This ensures the count is incremented correctly, regardless of how many times the function is invoked in quick succession.
Why Use the Functional Update Pattern?
The primary advantage of the Functional Update Pattern is its ability to access the most current state. This is particularly useful in situations with asynchronous state updates. For example, when multiple updates happen quickly one after another, directly referencing the state can lead to errors.
Another significant benefit is improved readability and maintainability. By isolating the logic for state updates, developers can easily follow how the state changes over time, which simplifies debugging. According to a survey by Stack Overflow, nearly 60% of developers believe that clarity in code enhances its maintainability.
Examples of the Functional Update Pattern in Action
Example 1: Incrementing a Counter
To further illustrate the Functional Update Pattern, consider a simple counter application where users can increment, decrement, and reset the count.
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => setCount(prevCount => prevCount + 1);
const decrement = () => setCount(prevCount => prevCount - 1);
const reset = () => setCount(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
<button onClick={reset}>Reset</button>
</div>
);
};
export default Counter;
With this implementation, the count updates correctly, even if buttons are clicked rapidly, demonstrating the reliability of the Functional Update Pattern.
Example 2: Managing a List of Users
The Functional Update Pattern excels when managing complex state structures, such as an array of items. This next example demonstrates how to handle a dynamic user list, where users can be added or removed easily.
import React, { useState } from 'react';
const UserList = () => {
const [users, setUsers] = useState([]);
const addUser = (name) => {
setUsers(prevUsers => [...prevUsers, name]);
};
const removeUser = (name) => {
setUsers(prevUsers => prevUsers.filter(user => user !== name));
};
return (
<div>
<h1>User List</h1>
<ul>
{users.map((user, index) => (
<li key={index}>{user}</li>
))}
</ul>
<button onClick={() => addUser('John Doe')}>Add John Doe</button>
<button onClick={() => removeUser('John Doe')}>Remove John Doe</button>
</div>
);
};
export default UserList;
In this case, using the Functional Update Pattern allows for reliable manipulation of the users array without fear of staleness.
Common Mistakes to Avoid
While the Functional Update Pattern offers many benefits, developers should avoid certain pitfalls:
Ignoring Previous State: Not using the previous state leads to inconsistent updates. Always leverage the previous state when making updates.
Non-Functional Updates in Quick Succession: If you run a non-functional update (`setCount(count + 1)`) during rapid state changes, you may end up with wrong results. The functional approach mitigates this issue.
Complex Logic: State updates should remain straightforward. Keep your functional updates clear and concise for better readability.
Performance Considerations
The Functional Update Pattern is efficient in many scenarios. However, knowing when to use it is crucial. For frequent updates, this pattern can enhance performance. Be cautious with deeply nested updates; if needed, consider using `useReducer` for better structure.
When rendering lists or components based on state, using tools like `React.memo` or `useMemo` can further optimize your application's performance.
Comparison with Other Patterns
The Functional Update Pattern is one of several state management strategies in React. Others include:
Direct State Updates: This method is suitable for simple situations but may result in stale state issues.
useReducer Hook: This is ideal for complex state logic, particularly when the next state relies on the previous state.
Context API: This provides a way to share state across components but can complicate smaller state slices.
The Functional Update Pattern often strikes a balance between simplicity and reliability, making it a favored choice for managing state at the component level.
Key Takeaways for Effective State Management
The Functional Update Pattern is a vital tool in the React developer's repertoire. By ensuring that state updates are reliable and easy to trace, you can create cleaner and more maintainable code. As you develop applications, remember how crucial this pattern is in enhancing state management.
By embracing the Functional Update Pattern, you can create React components that respond predictably and perform consistently. As you build more complex applications, continue to iterate and refine your use of this pattern for improved performance and clarity. Happy coding!
Why Use Functional Updates in setState?
Ever faced stale state issues in React?
Updating state incorrectly can lead to unexpected bugs. Let’s explore the functional update pattern in setState & why it matters!
The Problem: When updating state based on the previous state, doing it wrongly can cause issues.
Example of a risky state update:
setAvailableIngredients(availableIngredients.map((ingredient) =>
ingredient.id === id
? { ...ingredient, available: !ingredient.available }
: ingredient
));
This might not use the latest state if multiple updates happen quickly!
Why is this a problem? React batches state updates for performance, meaning availableIngredients may not be up to date when setState runs.
This can cause stale or inconsistent updates, especially in event handlers & async operations.
Why is This Important?
If we did something like this:
setAvailableIngredients(availableIngredients.map(...)); // ❌ Potential stale state issue
React might use an outdated state (availableIngredients might not be the latest).
The Solution: Functional Updates!
To ensure we always work with the latest state, pass a function to setState:
setAvailableIngredients((prevIngredients) =>
prevIngredients.map((ingredient) =>
ingredient.id === id
? { ...ingredient, available: !ingredient.available }
: ingredient
)
);
prevIngredients always contains the latest state.
By using:
setAvailableIngredients((prevIngredients) => { ... }); Note: we guarantee React always updates based on the latest state.
Functional Update Pattern: Here’s how it follows the functional update pattern:
Instead of setting availableIngredients directly, we pass a function to setAvailableIngredients.
This function receives prevIngredients (the latest state value).
It ensures that the update is based on the most up-to-date state, preventing stale state issues when multiple updates happen rapidly.
Why Functional Updates?
Prevents stale state issues when multiple updates happen in quick succession.
Ensures state changes are based on the latest data.
Recommended for updates that depend on previous state values.
Key Takeaways:
Always use functional updates in setState when the new state depends on the previous one.
Avoid directly using state inside setState when updating sequentially.
Improves performance & predictability in complex UIs.
Final Thoughts Using the functional update pattern in setState ensures your React app handles state updates correctly.
Comentários