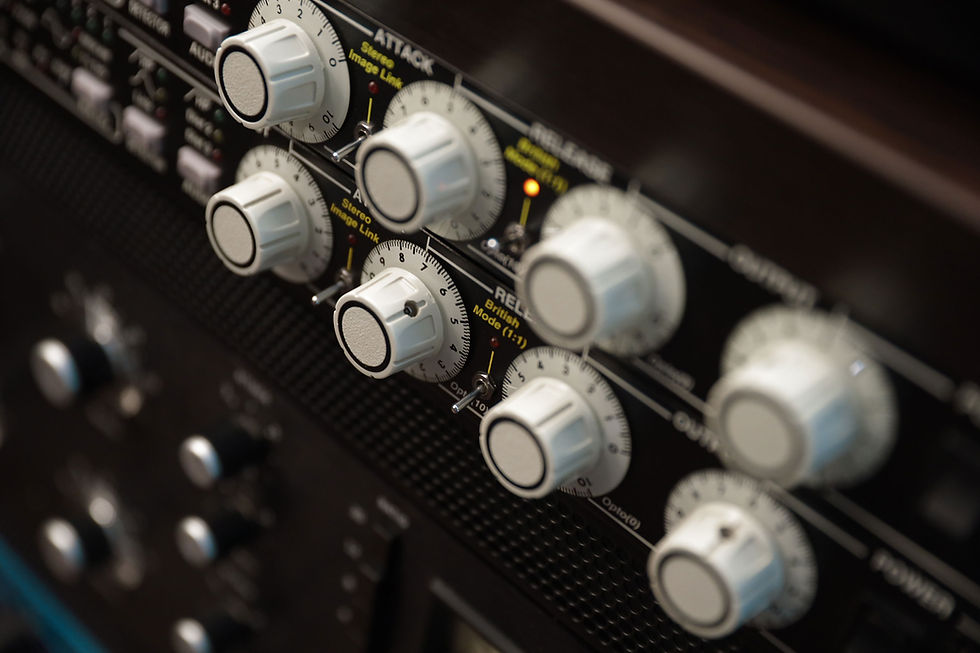
The controller module in a Node.js application is a part of the MVC architecture (Model-View-Controller) used to separate the business logic from other layers. Controllers handle requests from the client, interact with the models (data layer), and return appropriate responses.
A a controller is a module in Node.js application which helps us to separate concerns and build scalable applications. Controllers can manipulate data from the database or other sources to prepare it for presentation to the client. Controllers can implement business logic specific to the application, such as authentication, authorization, and validation. Controllers can act as middleware in the request-response cycle, performing tasks such as parsing request bodies, handling errors, and sending responses.
A controller typically exports one or more functions that correspond to different operations (e.g., CRUD operations).
project/
├── controllers/
│ └── userController.js
├── models/
│ └── userModel.js
├── routes/
│ └── userRoutes.js
├── server.js
Role of a Controller
Process Requests: Extract data from HTTP requests (e.g., parameters, query strings, and body).
Interact with Models: Fetch, insert, update, or delete data using the model layer.
Respond to Clients: Send appropriate responses back to the client (JSON, HTML, etc.).
Middleware Logic: Perform validations, handle errors, or delegate tasks to services.
Advantages of Using Controllers
Separation of Concerns: Keeps route definitions clean and concise.
Reusability: Business logic is reusable across different routes.
Scalability: New features can be added without disrupting existing functionality.
Maintainability: Isolated code is easier to debug and test.
Controller to handle requests for different routes in the application
In the code given below, handles requests for different routes in the application. For example, if a user makes a GET request to "/contacts", the ContactController can handle the request and return a list of contacts.
const asyncHandler = require("express-async-handler");
const Contact = require("../models/contactModel");
//@desc Get all contacts
//@route GET /api/contacts
//@access public
const getContacts = asyncHandler(async (req, res) => {
const contacts = await Contact.find();
res.status(200).json(contacts);
});
//@desc Create new contact
//@route POST /api/contacts
//@access public
const createContact = asyncHandler(async (req, res) => {
const {name, email, phone} = req.body;
if(!name || !email || !phone) {
res.status(400);
throw new Error("All Fileds Are Mandatory!");
}
const contact = await Contact.create({
name,
email,
phone,
})
res.status(200).json(contact);
});
//@desc Get contact
//@route GET /api/contacts/:id
//@access public
const getContact = asyncHandler(async (req, res) => {
const contact = await Contact.findById(req.params.id);
if(!contact){
res.status(404);
throw new Error("Contact Not Found!");
}
res.status(200).json(contact);
});
//@desc Update contact
//@route PUT /api/contacts/:id
//@access public
const updateContact = asyncHandler(async (req, res) => {
const contact = await Contact.findById(req.params.id);
if(!contact){
res.status(404);
throw new Error("Contact Not Found!");
}
const updatedContact = await Contact.findByIdAndUpdate(
req.params.id,
req.body,
{new: true}
)
res.status(200).json(updatedContact);
});
//@desc Delete contact
//@route DELETE /api/contacts/:id
//@access public
const deleteContact = asyncHandler(async (req, res) => {
const contact = await Contact.findById(req.params.id);
if(!contact){
res.status(404);
throw new Error("Contact Not Found!");
}
await Contact.findByIdAndRemove(req.params.id);
res.status(200).json({message:`Contact is deleted for ${req.params.id}`});
});
module.exports= { getContacts, createContact, getContact, updateContact,
deleteContact};
Comments