Context API React JS
- coding z2m
- Nov 3, 2024
- 4 min read
Updated: Jan 27
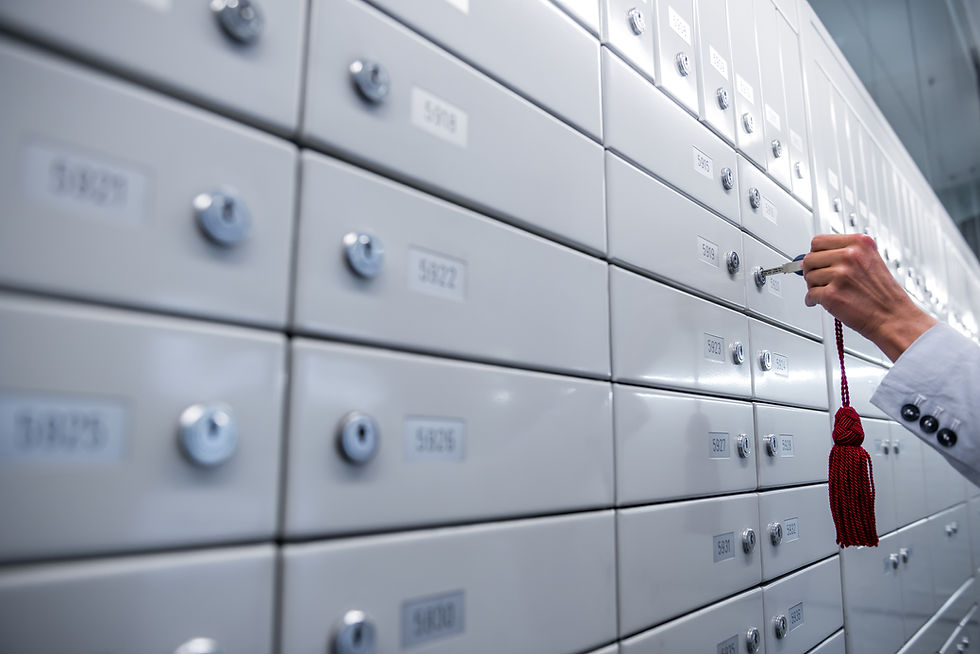
Context API React JS
What is the React Context API?
Context API React JS: The React Context API is a feature that enables data to be shared across components in a React application without the need to pass props down manually through every level of the component tree. This helps avoid “prop drilling,” where data has to be passed down through multiple nested components even if only the deepest component needs it.
How the React Context API Works
Create a Context: You start by creating a context using React.createContext(). This context will hold the data you want to share.
Provide the Context: Use the context’s Provider component to wrap parts of the component tree where you want the data to be accessible. The Provider component takes a value prop, which contains the data you wish to pass down.
Consume the Context: Any component within the Provider can access the data by using React.useContext() with the context itself, eliminating the need to pass the data as props through intermediate components.
Example of the React Context API
Imagine an e-commerce app where multiple components (like ProductList, Cart, and Checkout) need access to data about the currency symbol and product details. Using Context API, you can create a StoreContext that provides currency, products, and other relevant data to all nested components.
This API is ideal for situations where global data (like themes, user authentication status, or configurations) needs to be accessed in various parts of an application.
This code sets up a context for managing and sharing store-related data across a React application, using the React Context API. Let’s break it down step-by-step:
Example: import { createContext, useState } from "react";
import {products} from '../assets/assets'
export const StoreContext = createContext();
const StoreContextProvider = (props) => {
const currency = '$';
const deliveryFee = 10;
const [search, setSearch] = useState('');
const [showSearchBar, setShowSearchBar] = useState(false);
const value = {
products,
currency,
deliveryFee,
search,
setSearch,
showSearchBar,
setShowSearchBar,
}
return (
<StoreContext.Provider value={value}>
{props.children}
</StoreContext.Provider>
)
}
export default StoreContextProvider;
1. Import Statements
import { createContext } from "react"; import { products } from '../assets/assets';
2. Creating the Context export const StoreContext = createContext(); StoreContext: This line creates a new context and exports it. Other components can access this context to consume data or be wrapped in the provider component. 3. Defining the Context Provider Component const StoreContextProvider = (props) => {
const currency = '$';
const deliveryFee = 10;
const value = {
products,
currency,
deliveryFee
};
return (
<StoreContext.Provider value={value}>
{props.children}
</StoreContext.Provider>
);
};
StoreContextProvider: This is a provider component that makes StoreContext data available to all components wrapped within it.
currency and deliveryFee: Define static values for currency and deliveryFee, which will be accessible through the context.
value: An object holding products, currency, and deliveryFee. This will be passed down to all child components.
The StoreContext.Provider component wraps {props.children}, which represents any child components within this provider in the component tree.
4. Exporting the Provider
export default StoreContextProvider;
By exporting StoreContextProvider, other components in the app can use it to wrap their JSX, enabling access to the shared context data (products, currency, deliveryFee) throughout the component tree.
Usage in Other Components
Any component within the StoreContextProvider wrapper can access products, currency, and deliveryFee by using useContext(StoreContext). This allows components to easily access store-related data without needing to manually pass it down as props.
How the Provider Works
The Provider component for a context (here, StoreContext.Provider) is a special component that React provides when you create a context with createContext(). This Provider is part of the StoreContext itself, and it’s used to wrap any child components that need access to the context's values.
In this line:
<StoreContext.Provider value={value}>
{props.children}
</StoreContext.Provider>
StoreContext.Provider: This is the Provider for the StoreContext, which makes the context’s value accessible to any components nested inside of it.
value={value}: This value prop is what the context will share with any components that consume it. Here, value contains { products, currency, deliveryFee }.
How StoreContext.Provider component wraps any child components?
n React, the StoreContext.Provider component wraps any child components by placing them inside the Provider as children, which gives those components access to the context's data. Here’s how this works in practice:
Inside StoreContextProvider
In the StoreContextProvider component, StoreContext.Provider wraps {props.children}, which represents any components nested within StoreContextProvider:
const StoreContextProvider = (props) => {
const currency = '$';
const deliveryFee = 10;
const value = {
products,
currency,
deliveryFee
};
return (
<StoreContext.Provider value={value}>
{props.children}
</StoreContext.Provider>
);
};
How {props.children} Works
props.children: This represents any child components that are passed to StoreContextProvider when it’s used in the application. When a component is wrapped in StoreContextProvider, it gets passed as props.children to StoreContextProvider.
Example of Wrapping Components
Suppose you have a component structure where you want to wrap multiple components within StoreContextProvider to share store data.
import StoreContextProvider from './path/to/StoreContextProvider';
import Header from './Header';
import ProductList from './ProductList';
import Cart from './Cart';
const App = () => {
return (
<StoreContextProvider>
<Header />
<ProductList />
<Cart />
</StoreContextProvider>
);
};
In this example:
Header, ProductList, and Cart are nested as child components within StoreContextProvider.
Inside StoreContextProvider, {props.children} renders Header, ProductList, and Cart within StoreContext.Provider.
Result: Context Data Access for All Children
Because StoreContextProvider uses StoreContext.Provider to wrap {props.children}, all child components within it (like Header, ProductList, and Cart) can access the StoreContext data through useContext(StoreContext). This structure is what makes the StoreContext data (like products, currency, and deliveryFee) available to all components nested within StoreContextProvider.
Where and How StoreContext Data is Accessed
Once StoreContextProvider wraps child components in the component tree, these children and their descendants can access StoreContext values using React's useContext hook, as shown below:
import React, { useContext } from 'react';
import { StoreContext } from './path/to/context';
const SomeComponent = () => {
const { products, currency, deliveryFee } = useContext(StoreContext);
return (
<div>
<p>Currency: {currency}</p>
<p>Delivery Fee: {deliveryFee}</p>
<ul>
{products.map(product => (
<li key={product.id}>{product.name}</li>
))}
</ul>
</div>
);
};
In this example:
useContext(StoreContext) is used to access StoreContext's value, which includes products, currency, and deliveryFee.
This data is available only because SomeComponent is a child (or descendant) of StoreContextProvider.
Comments